How to animate changes in a UICollectionView datasource in Swift
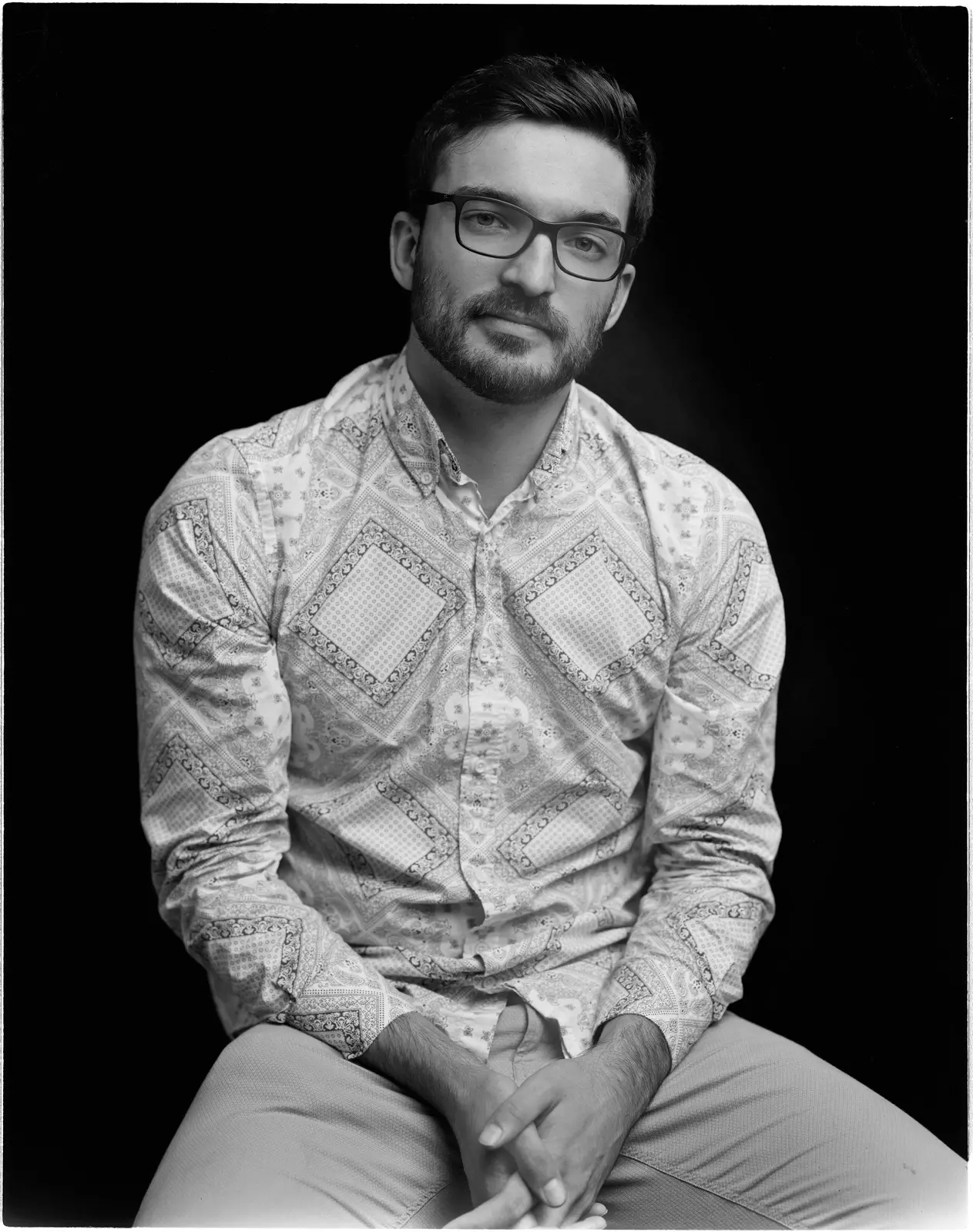
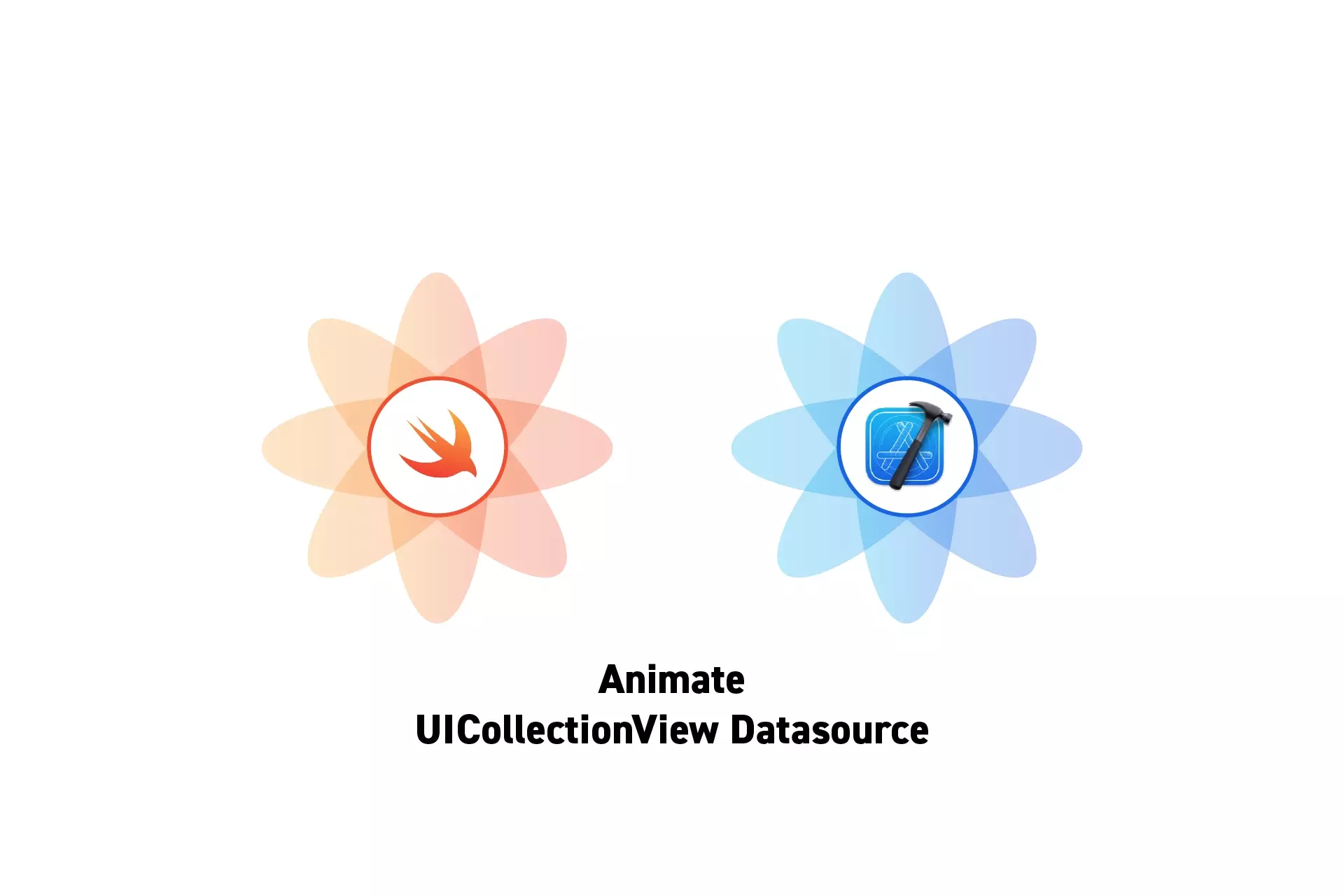
Update the datasource and then, use the insertItems and deleteItems functionality to animate changes in a UICollectionView datasource.
The following tutorial offers an Open Source Project that demonstrates how to animate changes in a UICollectionView datasource without using the reloadData function.
The code for this tutorial can be found in the repository linked below on the tutorial/uicollectionview/animate-datasource branch.
git clone git@github.com:delasign/swift-starter-project.git
Process
In order for you to animate changes in a UICollectionView datasource correctly you must first update the datasource and then insert or delete the necessary items at the relevant index paths.
If you insert or delete items before updating the datasource, you will get errors similar to:
- NSInteger index is negative or too large: -1.
- Invalid update: invalid number of items in section 0. The number of items contained in an existing section after the update must be equal to the number of items contained in that section before the update, plus or minus the number of items inserted or deleted from that section and plus or minus the number of items moved into or out of that section.
Functionality
The functions below can be used to insert and delete items as well as reloading items and sections.
Please note that reloading items and sections is not always necessary.
Insert Items
self.collectionView.insertItems(at: [ IndexPath(item: 1, section: 0), IndexPath(item: 2, section: 0), ... ])
Delete Items
self.collectionView.deleteItems(at: [ IndexPath(item: 1, section: 0), IndexPath(item: 2, section: 0), ... ])
Reload Items
self.collectionView.reloadItems(at: [IndexPath(item: 1, section: 0), IndexPath(item: 2, section: 0)], ...)
Reload Sections
self.collectionView.reloadSections(IndexSet(integersIn: 0...2))
Looking to learn more about things you can do with Swift and XCode ?
Search our blog to find educational content on learning how to use Swift and XCode.