How to add Orbit Controls to a ThreeJS scene in ReactJS
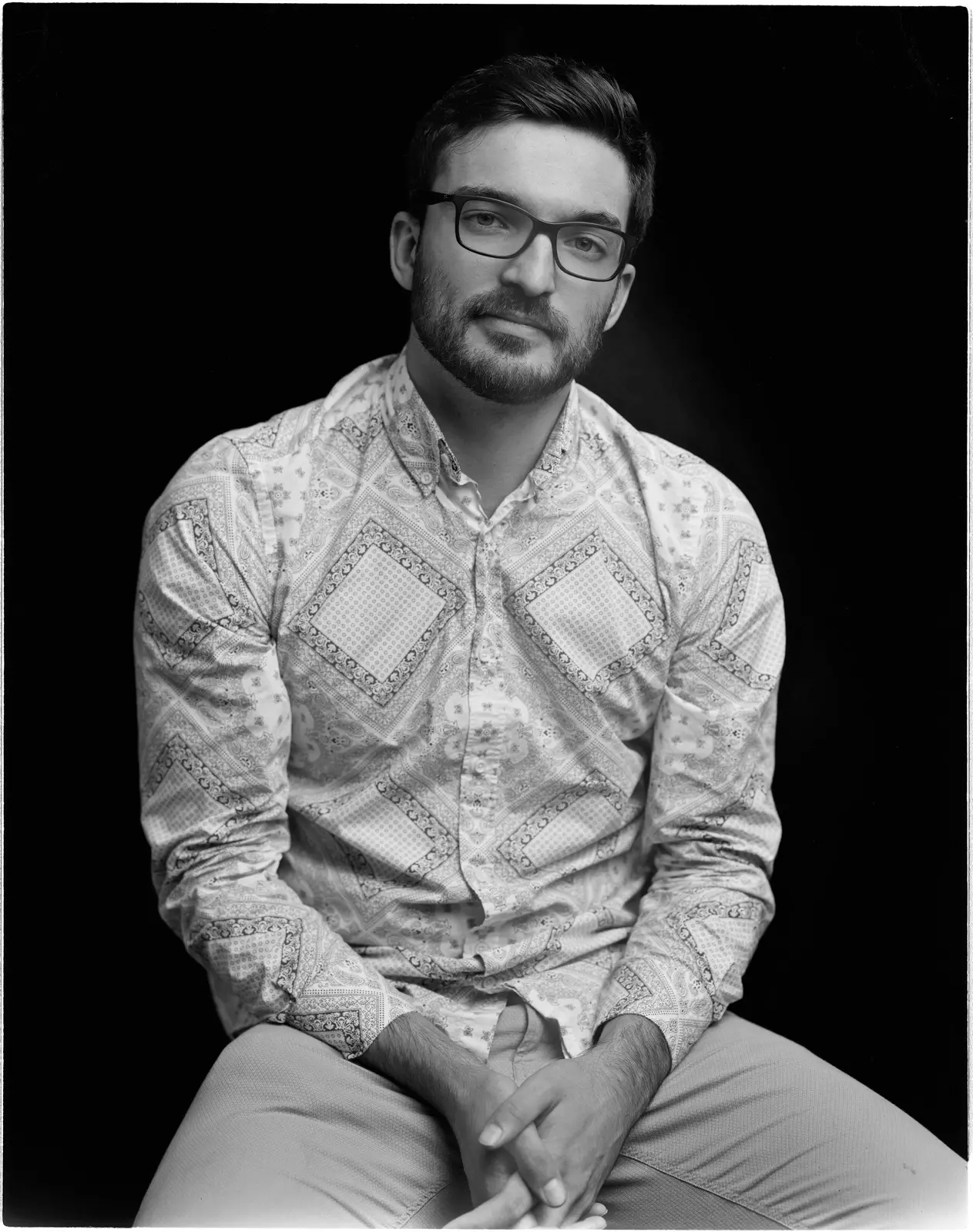
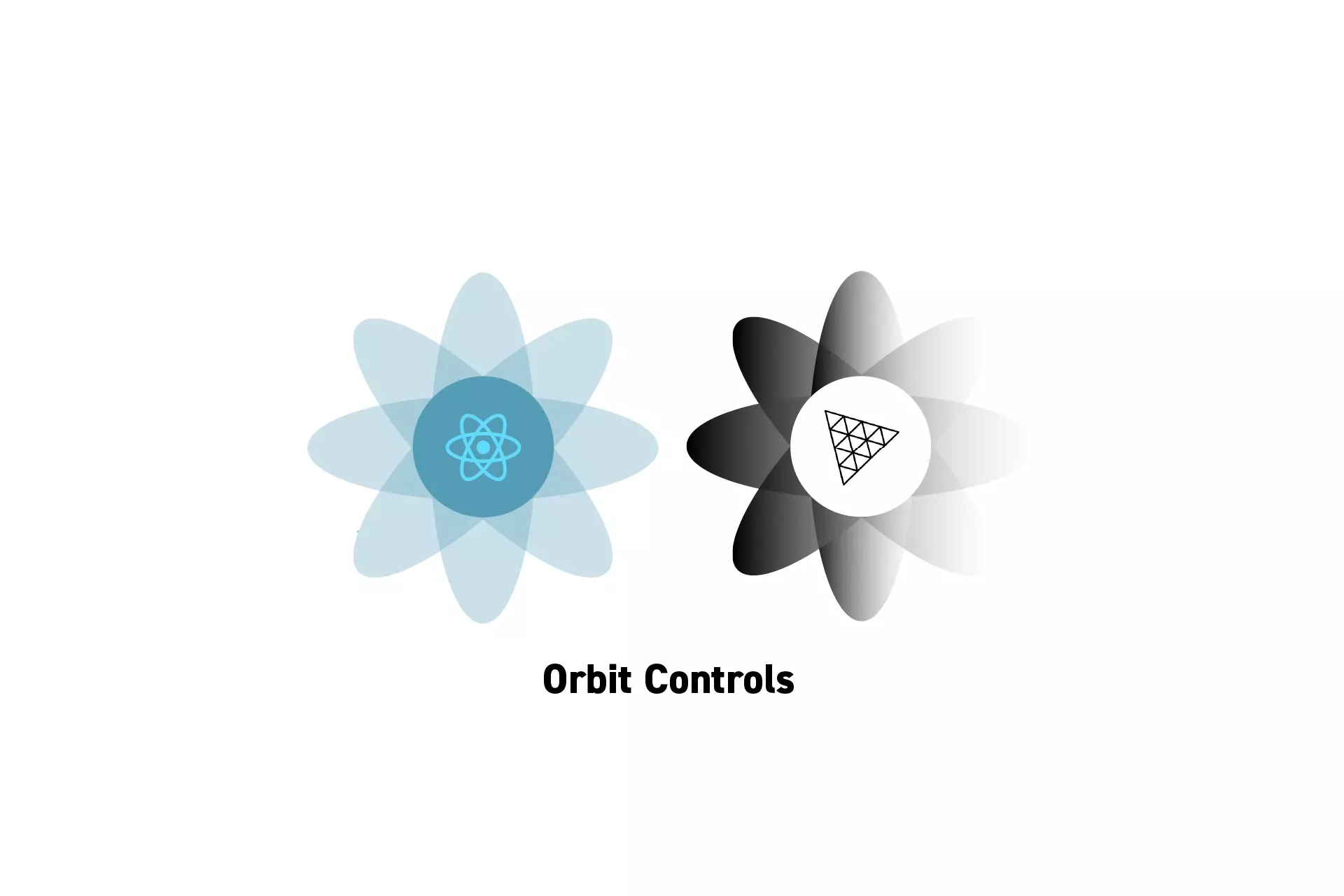
Import the Orbit Controls, set them up and update them in the animation frame.
We recommend that you clone our Open Source React-Redux Starter Project, checking out the tutorial/three-js/starter branch and carrying out the steps below. The changes can be found on the tutorial/three-js/orbital-controls branch.
git clone git@github.com:delasign/react-redux-starter-project.git
Step One: Setup the Project
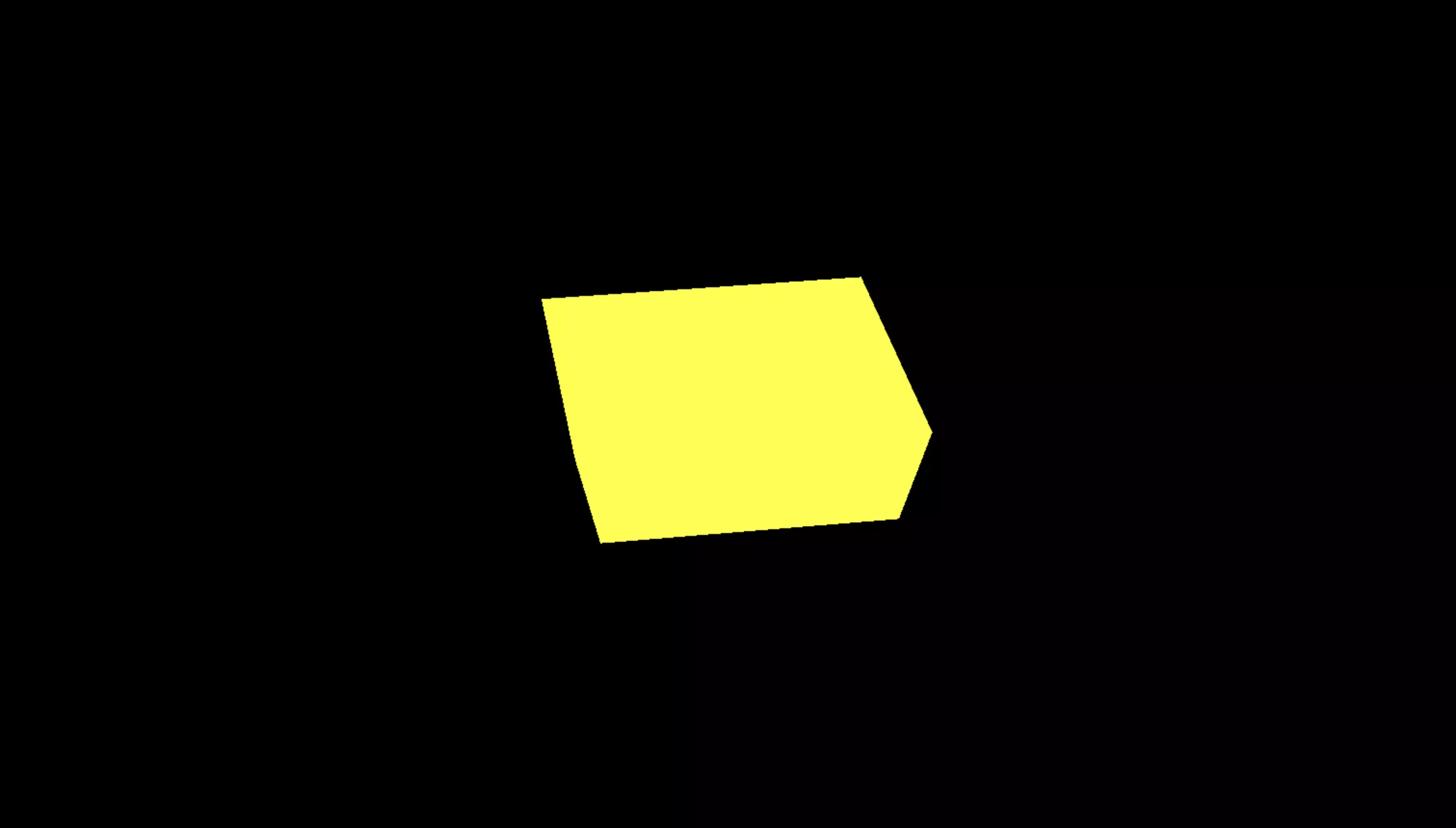
Follow the tutorial below to learn how to create a ThreeJS project that implements a shader on a box geometry.
Step Two: Import the Controls
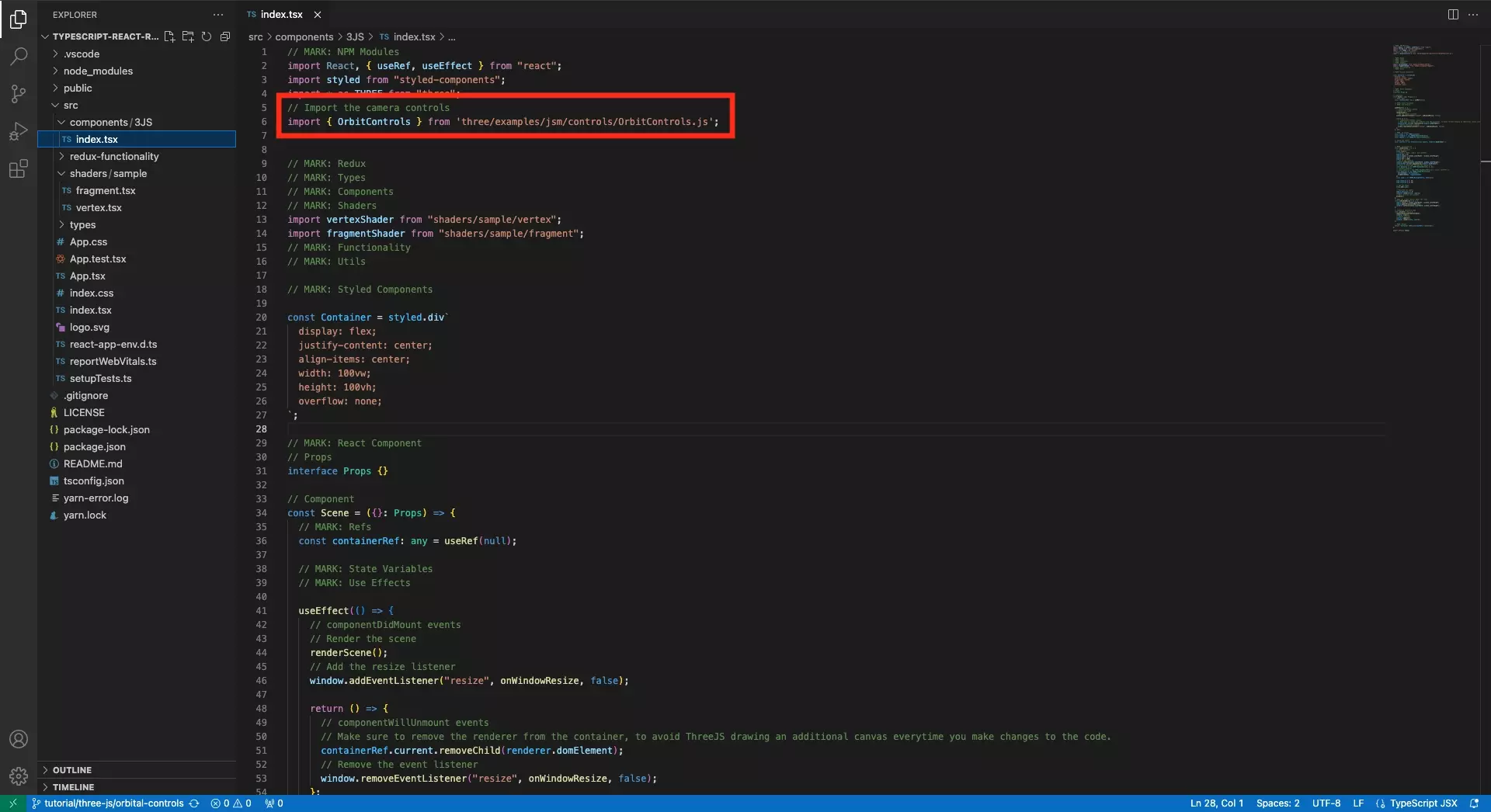
At the top of your file, import the Orbit Controls.
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls.js';
Step Three: Initialize the Controls
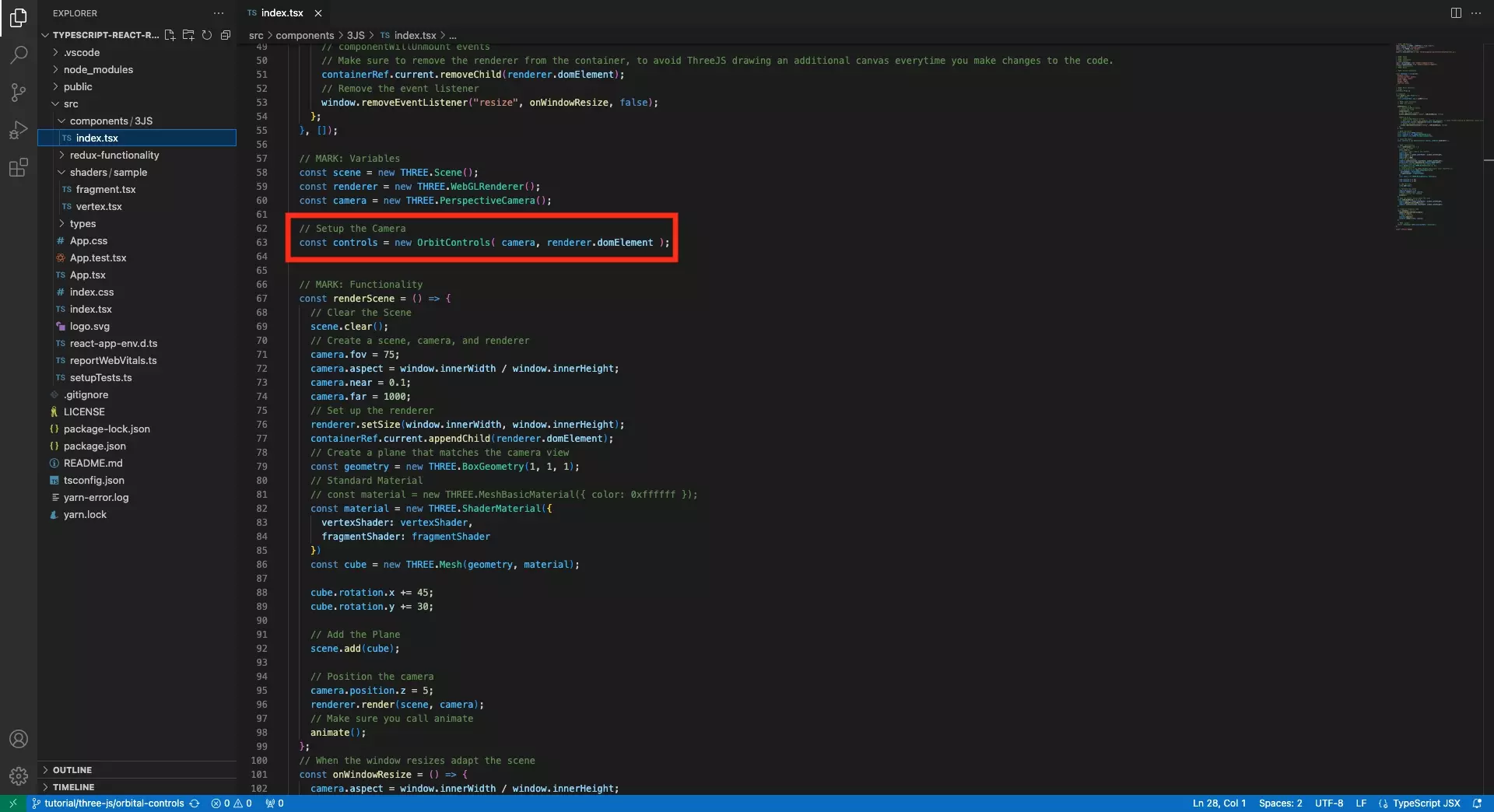
Within your react component, after declaring camera and renderer, initialize the Orbit Controls using code similar to the one below.
const controls = new OrbitControls( camera, renderer.domElement );
Step Four: Update Controls in Animation
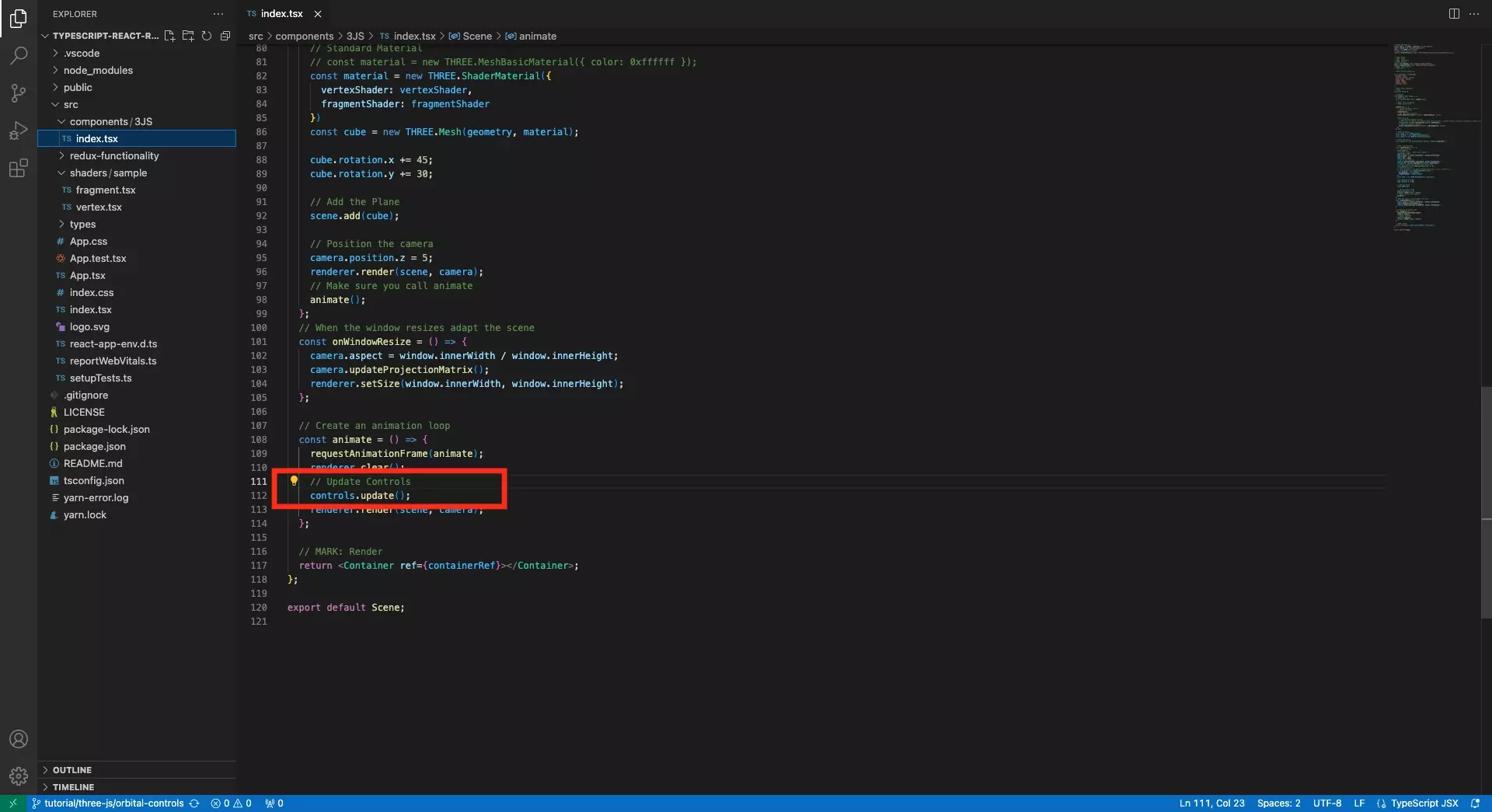
Within your animation loop, call the following function:
controls.update()
Step Five: Test
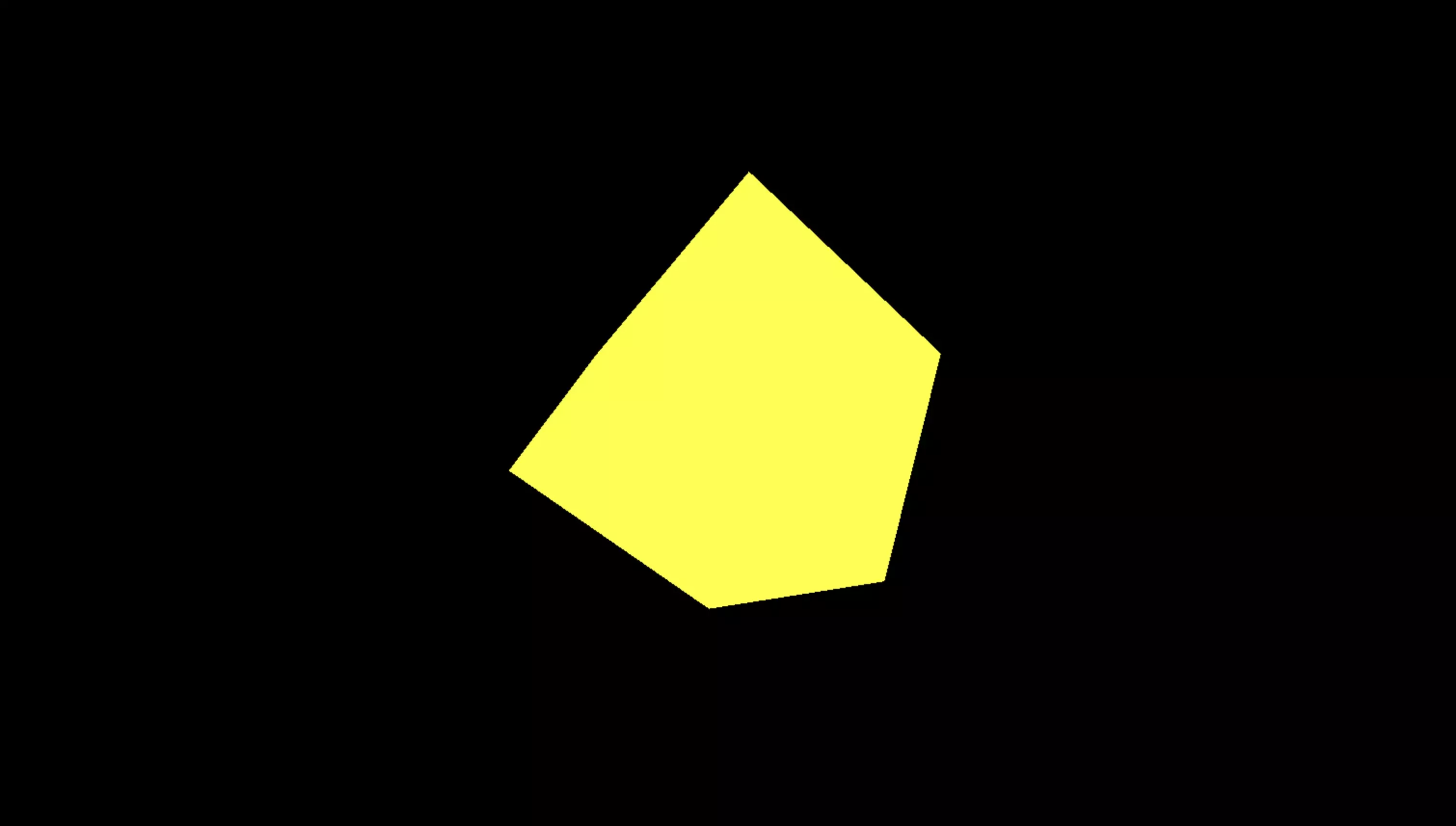
Run the code and confirm that you can now rotate, move and zoom in and out using your trackpad and keyboard.
Looking to learn more about ReactJS and ThreeJS ?
Search our blog to find educational content on learning how to use ReactJS and ThreeJS.