How to color the faces of a box geometry using a shader in ThreeJS
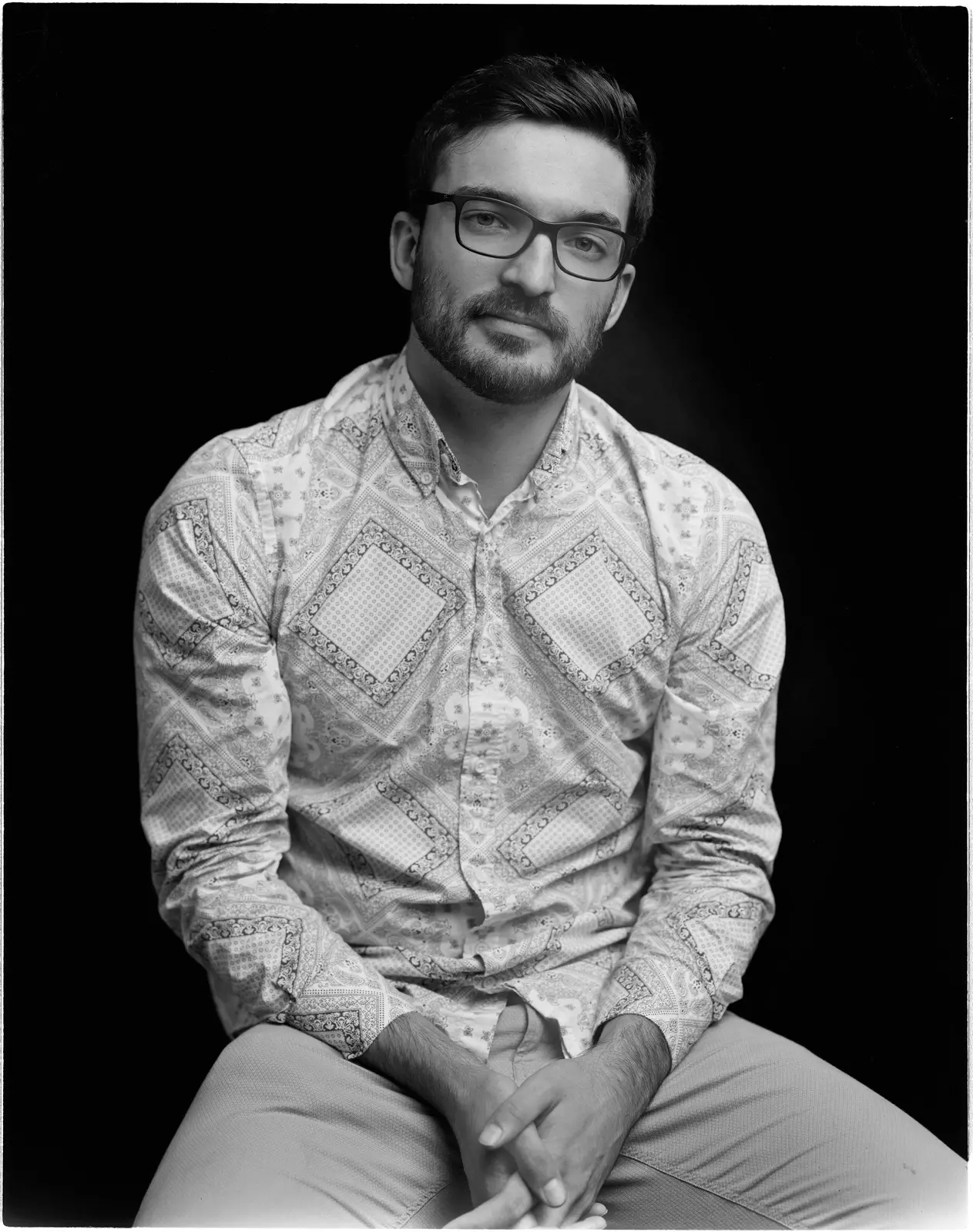
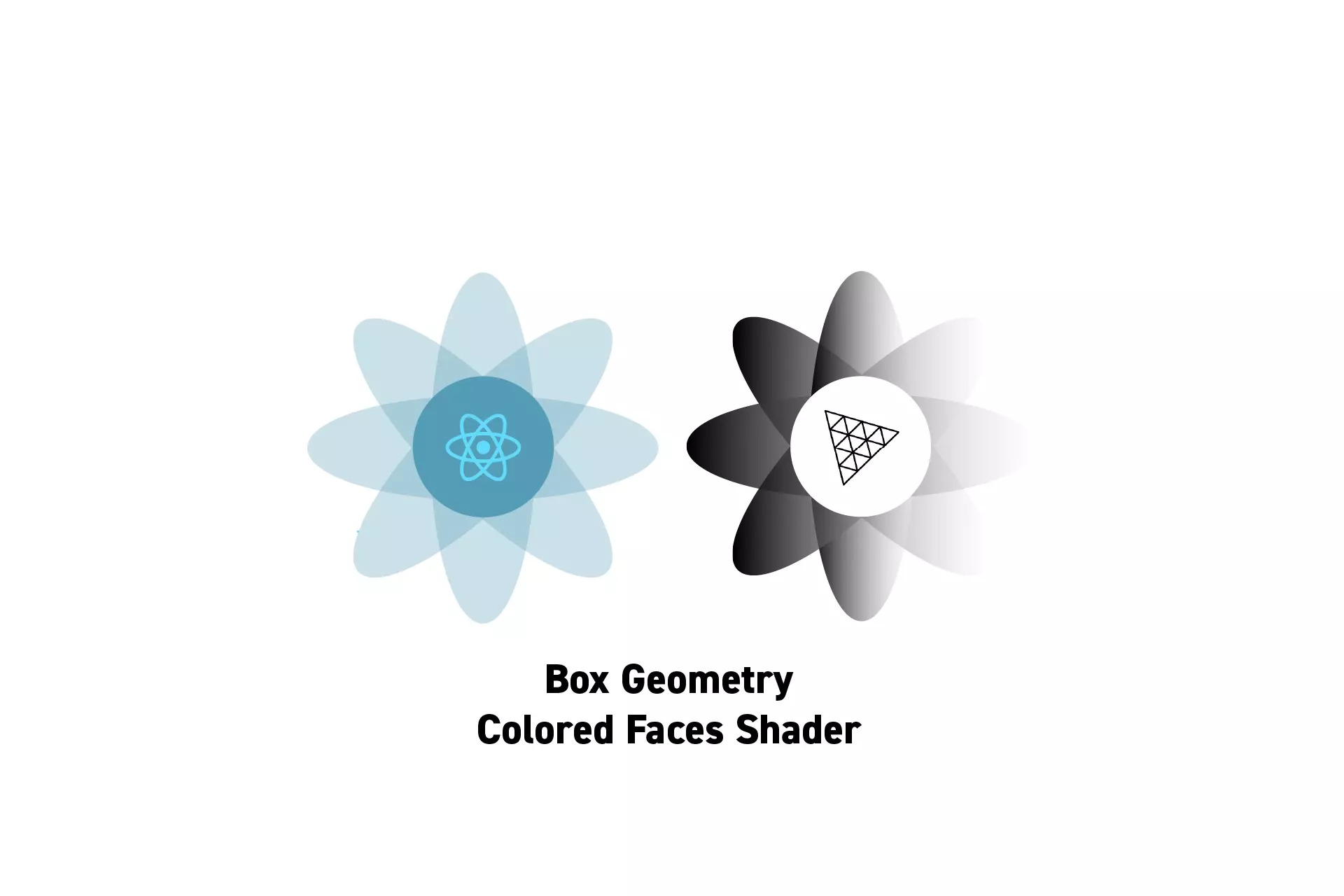
A step by step tutorial on coloring the faces of a box geometry using a shader in ThreeJS using ReactJS.
We recommend that you clone our Open Source React-Redux Starter Project, checking out the tutorial/three-js/starter branch and carrying out the steps below. The changes can be found on the tutorial/three-js/cube-colored-faces-shader-material branch.
Box Geometry Faces & Vertices
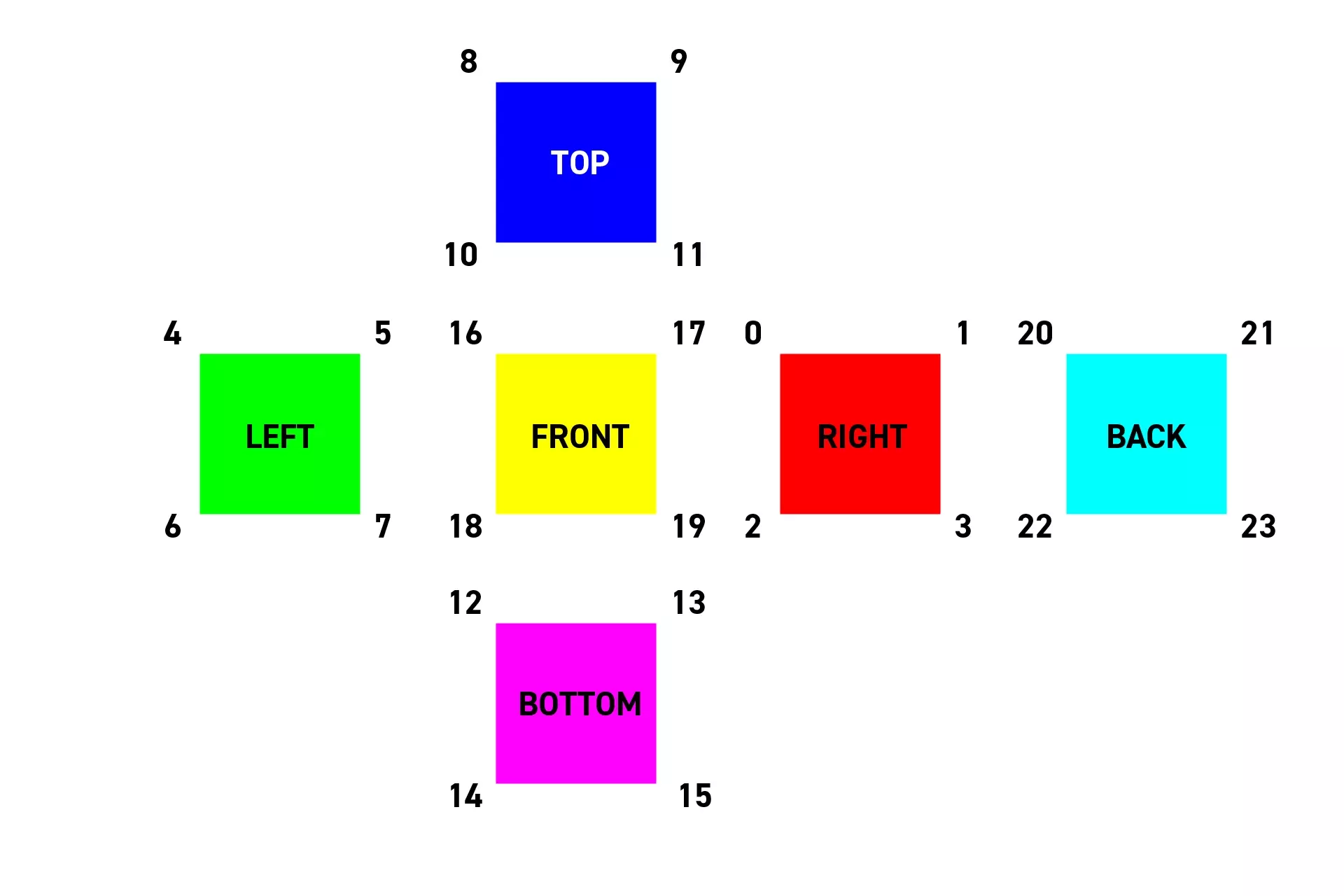
The diagram above describes what vertex applies to what face within a box geometry and the color that we applied to each face as part of this tutorial.
Tutorial
Step One: Setup the Project
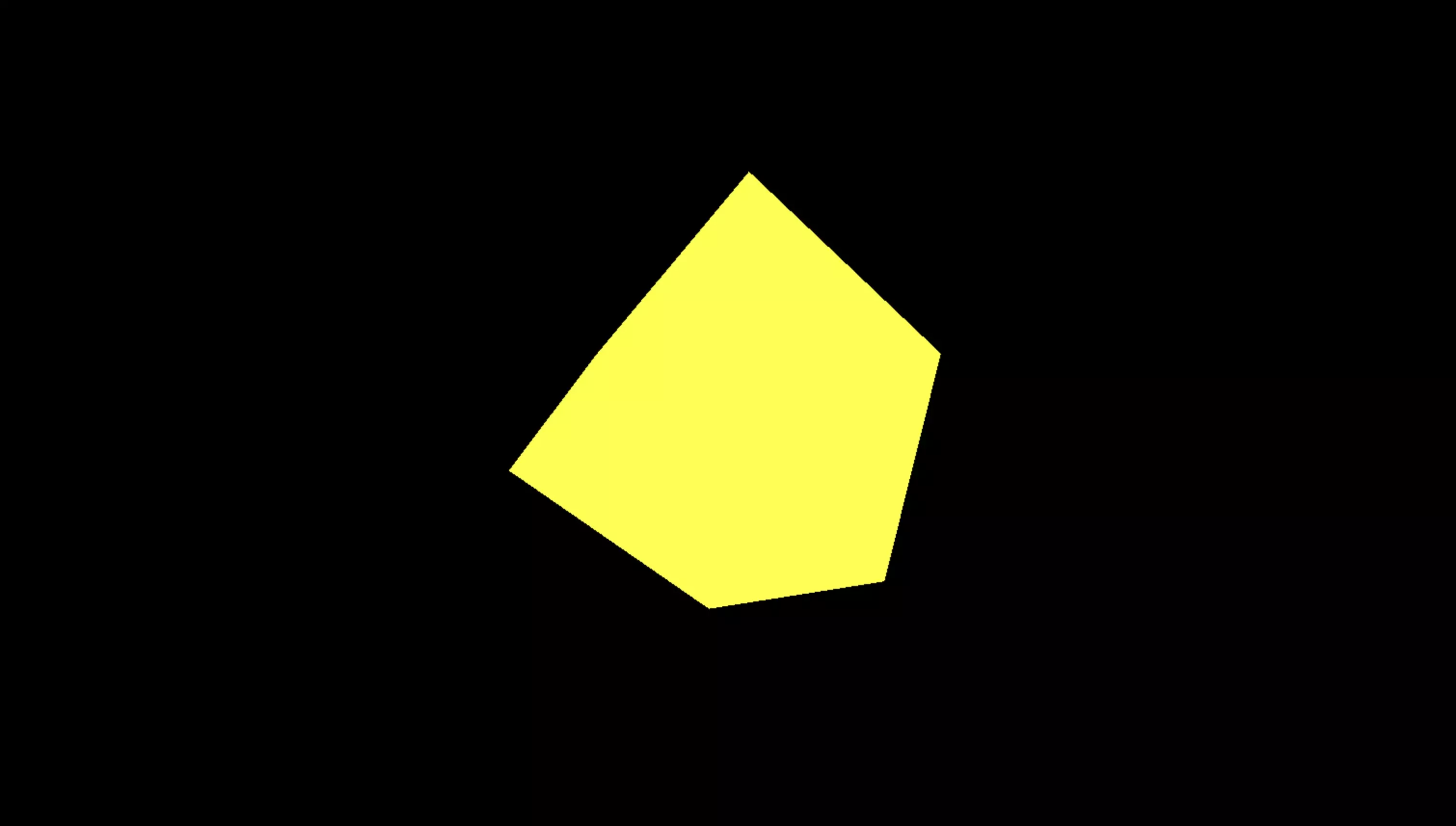
Follow the tutorials below to learn how to create a ThreeJS project that implements a shader on a box geometry, which can be explored using Orbit Controls.
Step Two: Set the Vertex Colors
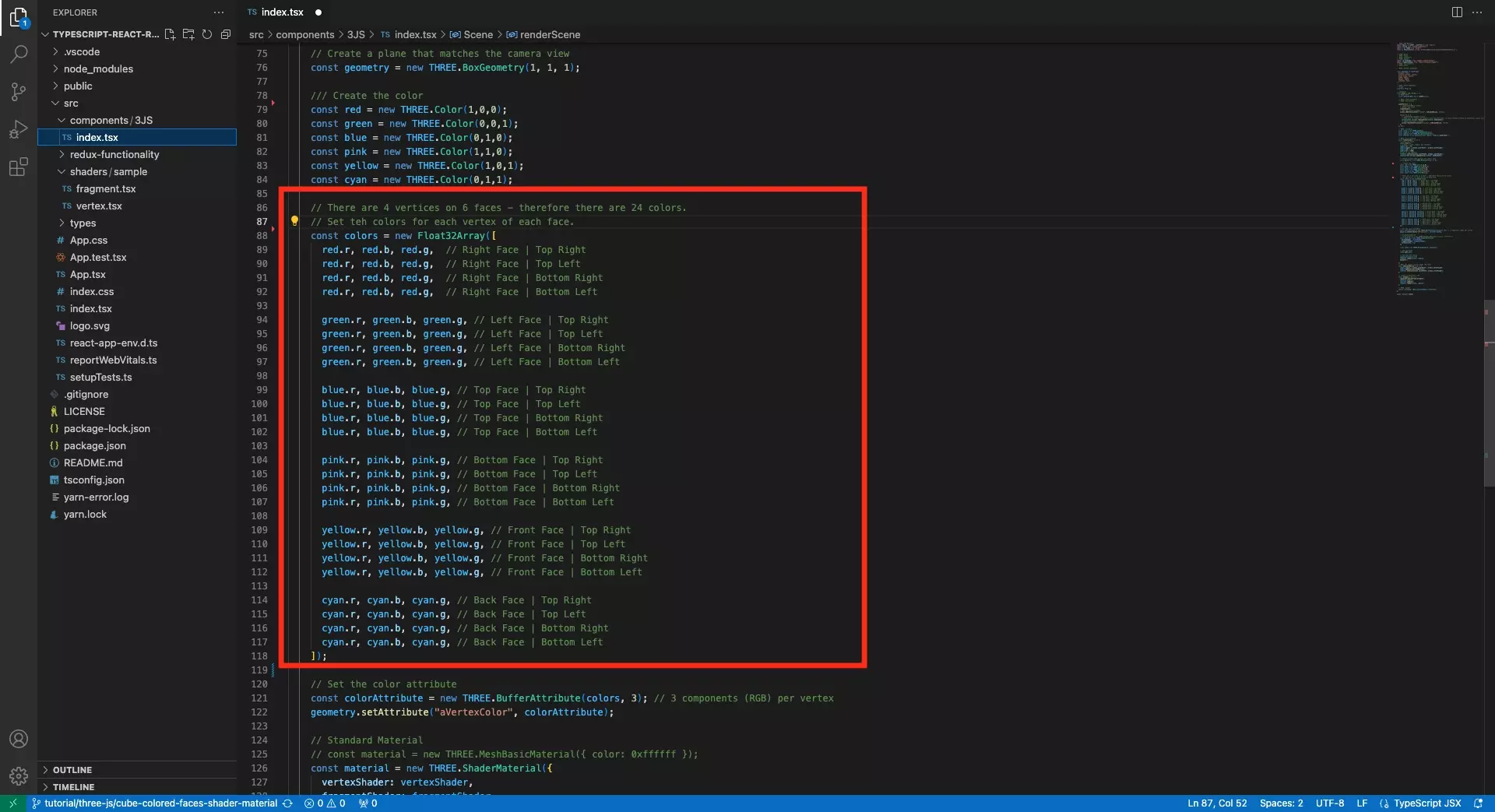
Create a Float32Array array that holds the 24 colors for the cube.
If you wish to set specific colors for each vertex, consult the diagram above the tutorial.
Step Three: Set the Color Attribute
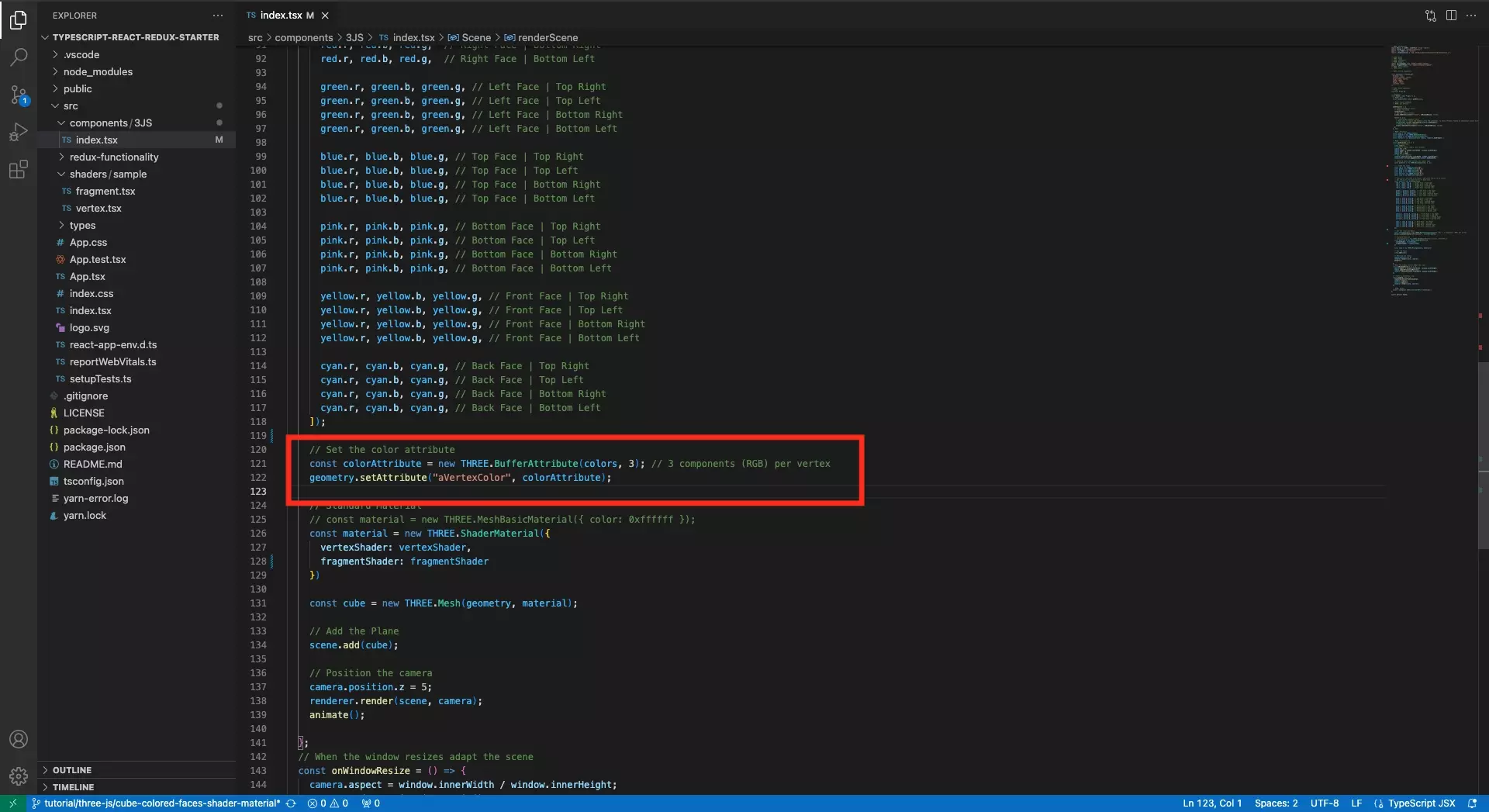
Apply the colors to the shader by setting them as an attribute using code similar to the one below.
const colorAttribute = new THREE.BufferAttribute(colors, 3); // 3 components (RGB) per vertex
geometry.setAttribute("aVertexColor", colorAttribute);
Step Four: Update Shaders
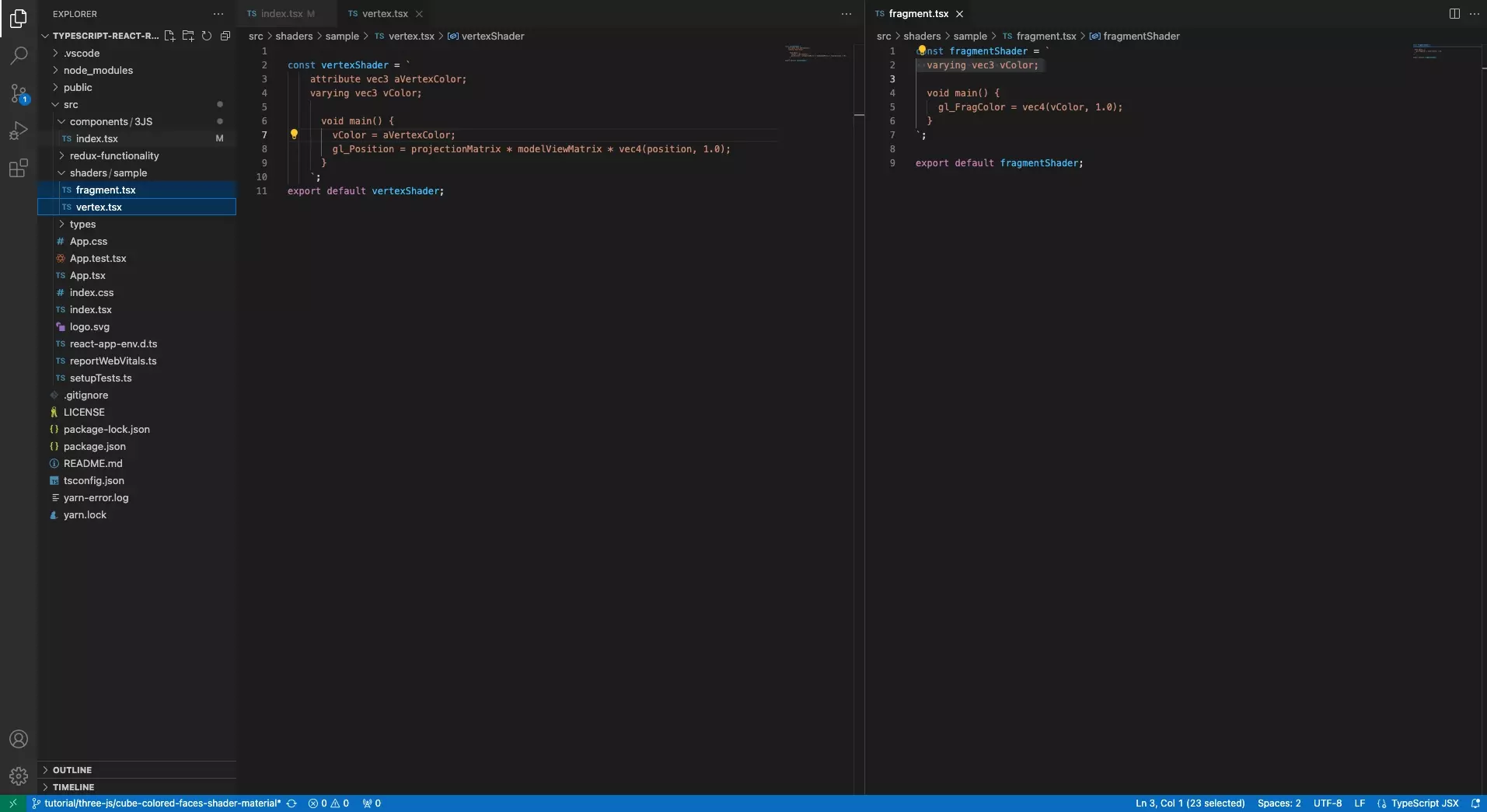
Update the vertex and fragment shader to work with the attribute.
Vertex Shader
Fragment Shader
Step Five: Test
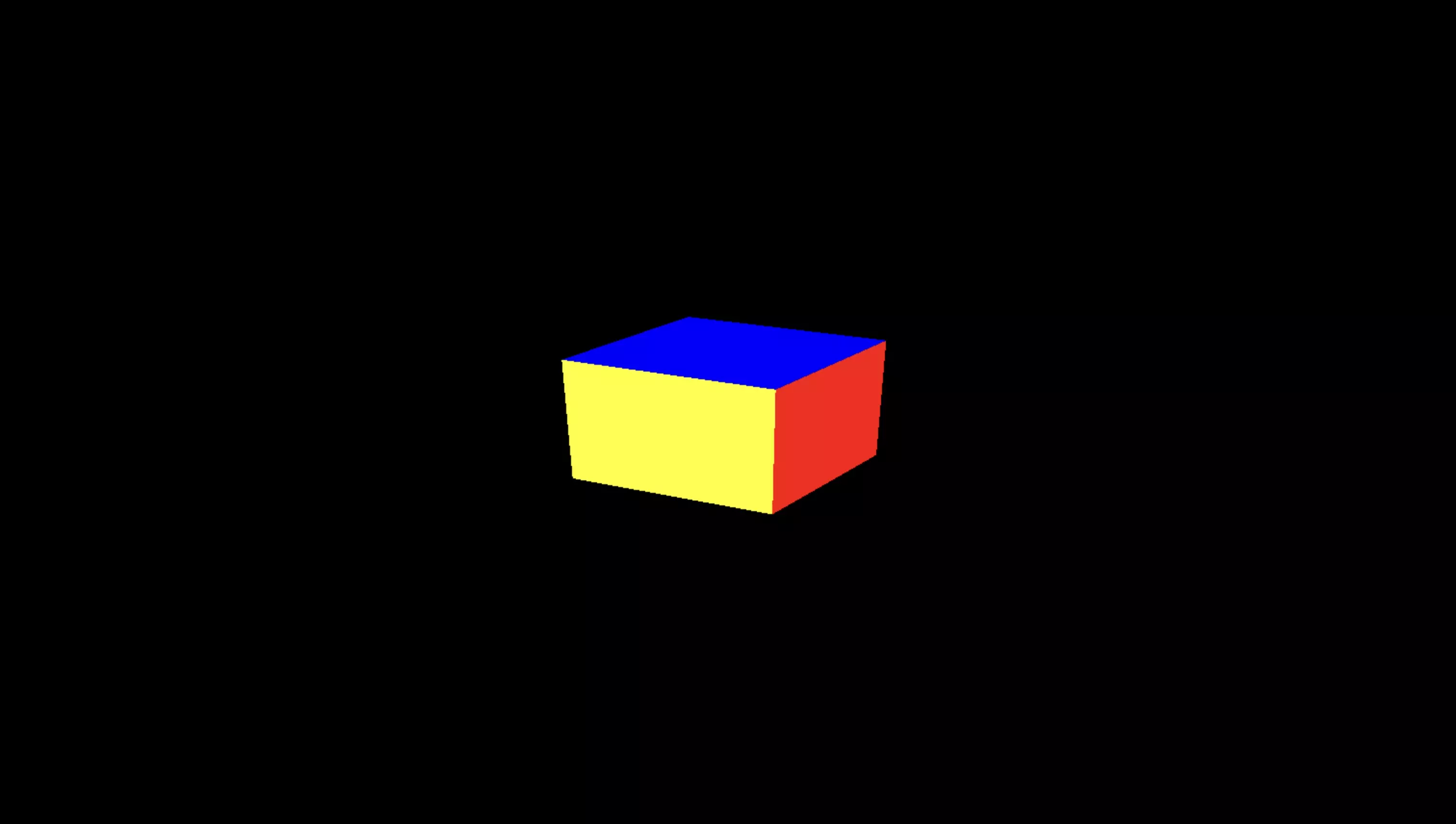
Run the code and confirm the shader works as expected.
Looking to learn more about ReactJS and ThreeJS ?
Search our blog to find educational content on learning how to use ReactJS and ThreeJS.