How to use dat.gui with ReactJS and ThreeJS
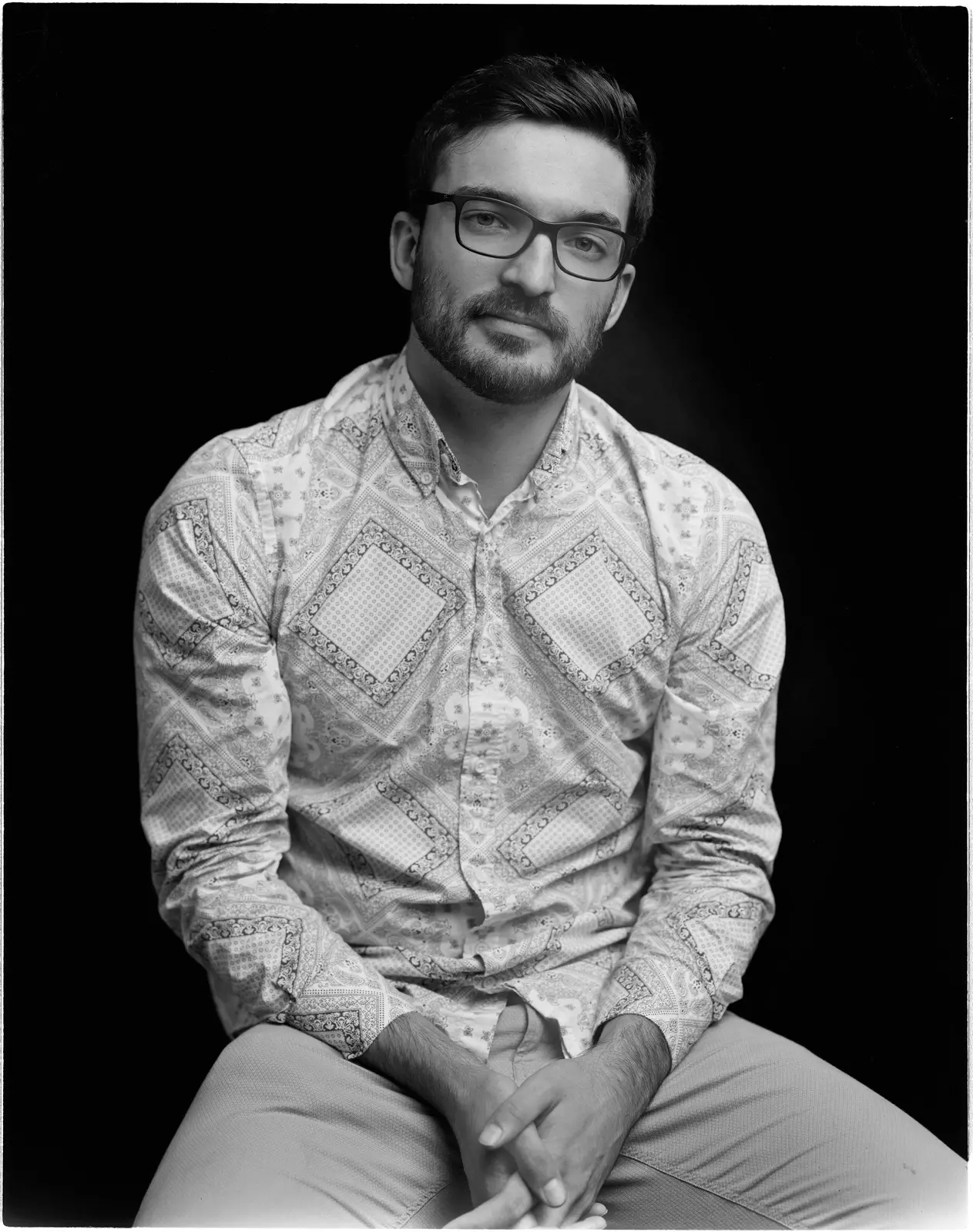
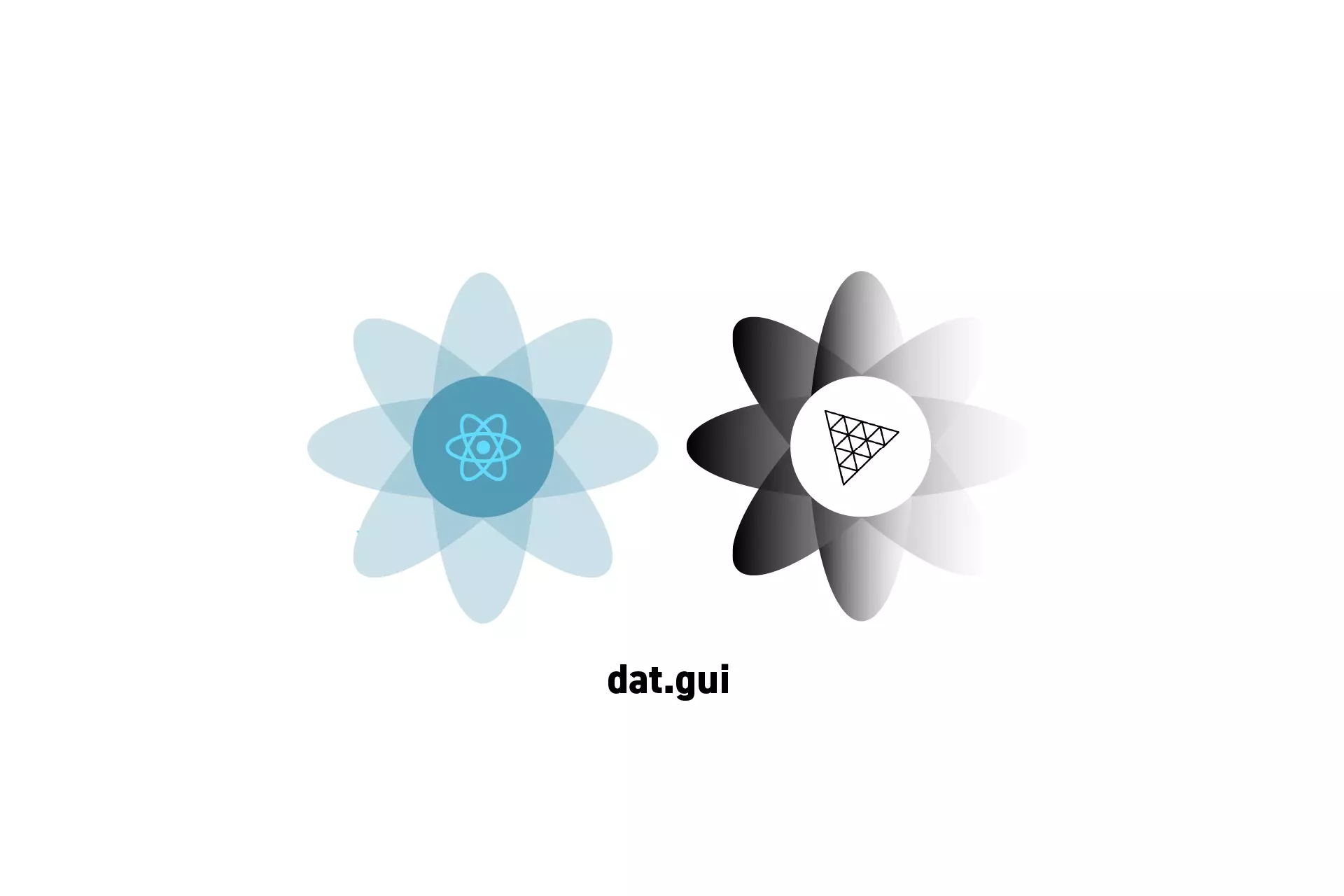
A step by step tutorial on creating UI that allows you to dynamically adapt a 3JS scene in realtime.
The proposed solution can be found on the main branch of our Open Source React, Typescript & ViteJS Starter Project.
git clone git@github.com:delasign/reactjs-3js-vitejs-starter-project.git
Step One: Setup the Project
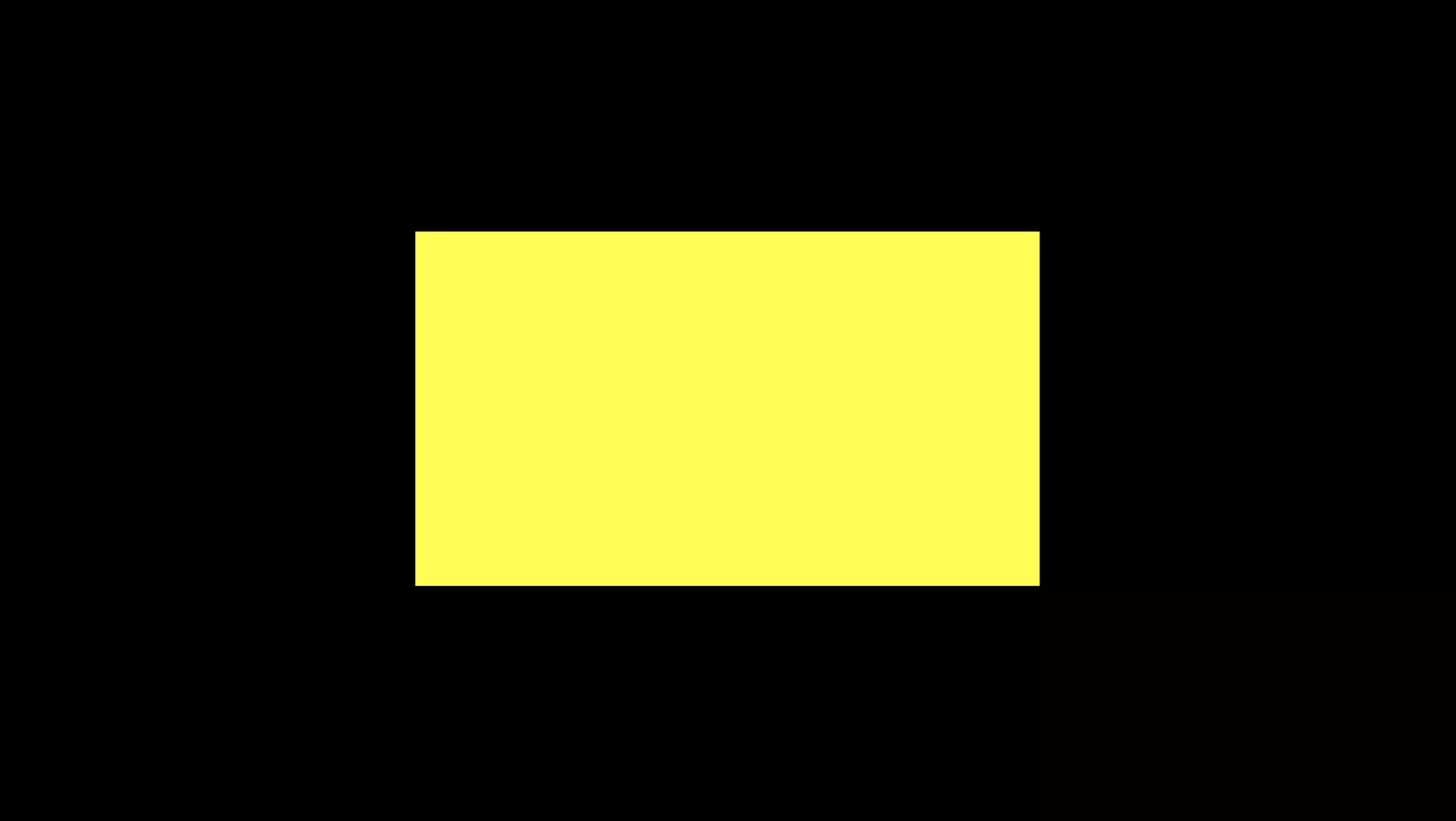
Follow the tutorial below to learn how to setup a Typescript & ViteJS project that works with a GLSL shader.
Step Two: Add Dependencies
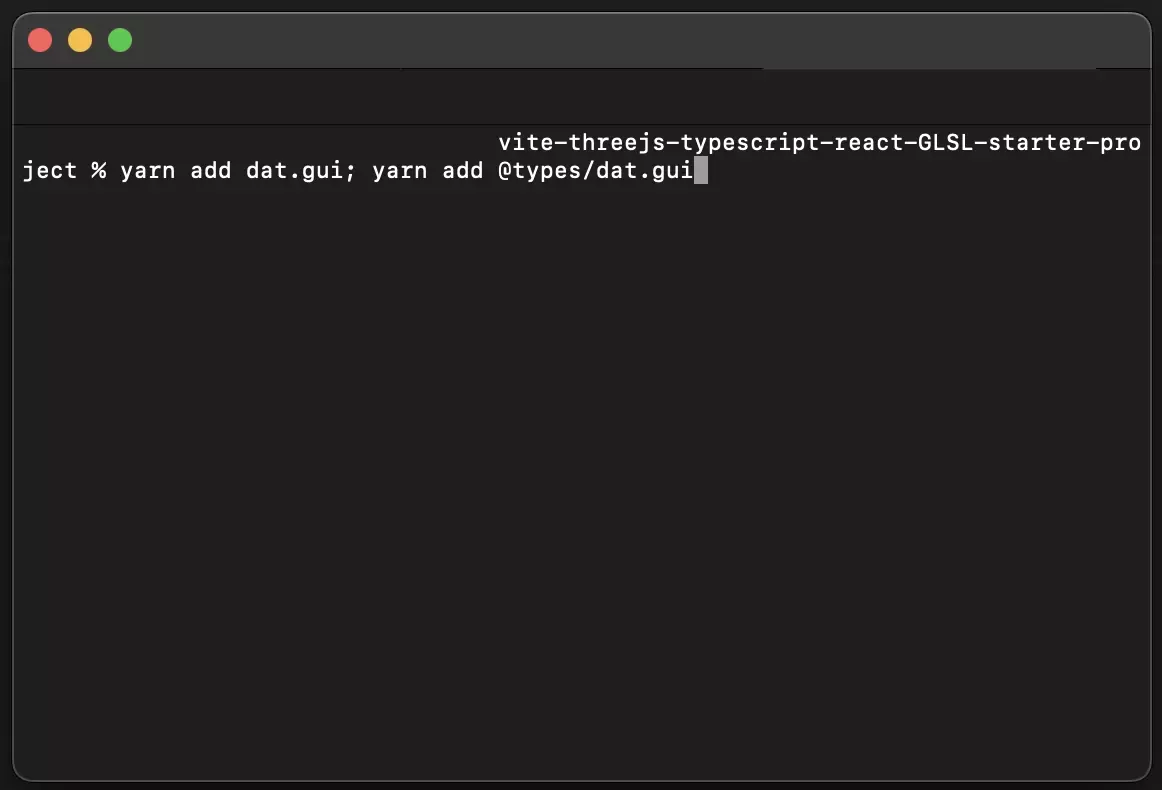
In Terminal, with the current directory set to that of the project, add the dat.gui dependency using the line below:
yarn add dat.gui; yarn add @types/dat.gui
Step Three: Import dat.gui
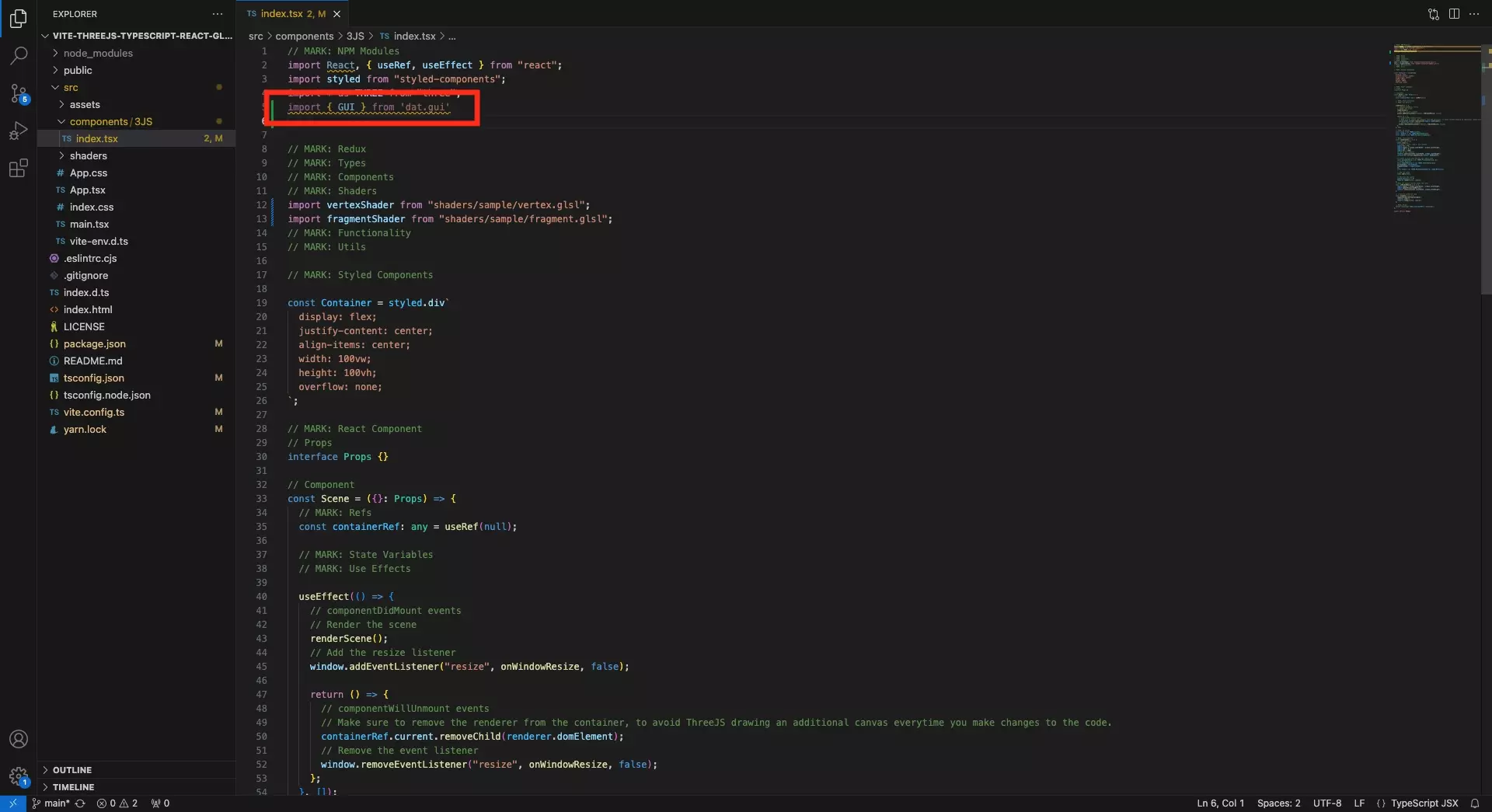
In the file that holds the ThreeJS scene, import dat.gui's component using the line below:
import { GUI } from 'dat.gui'
Step Four: Add GUI Constant
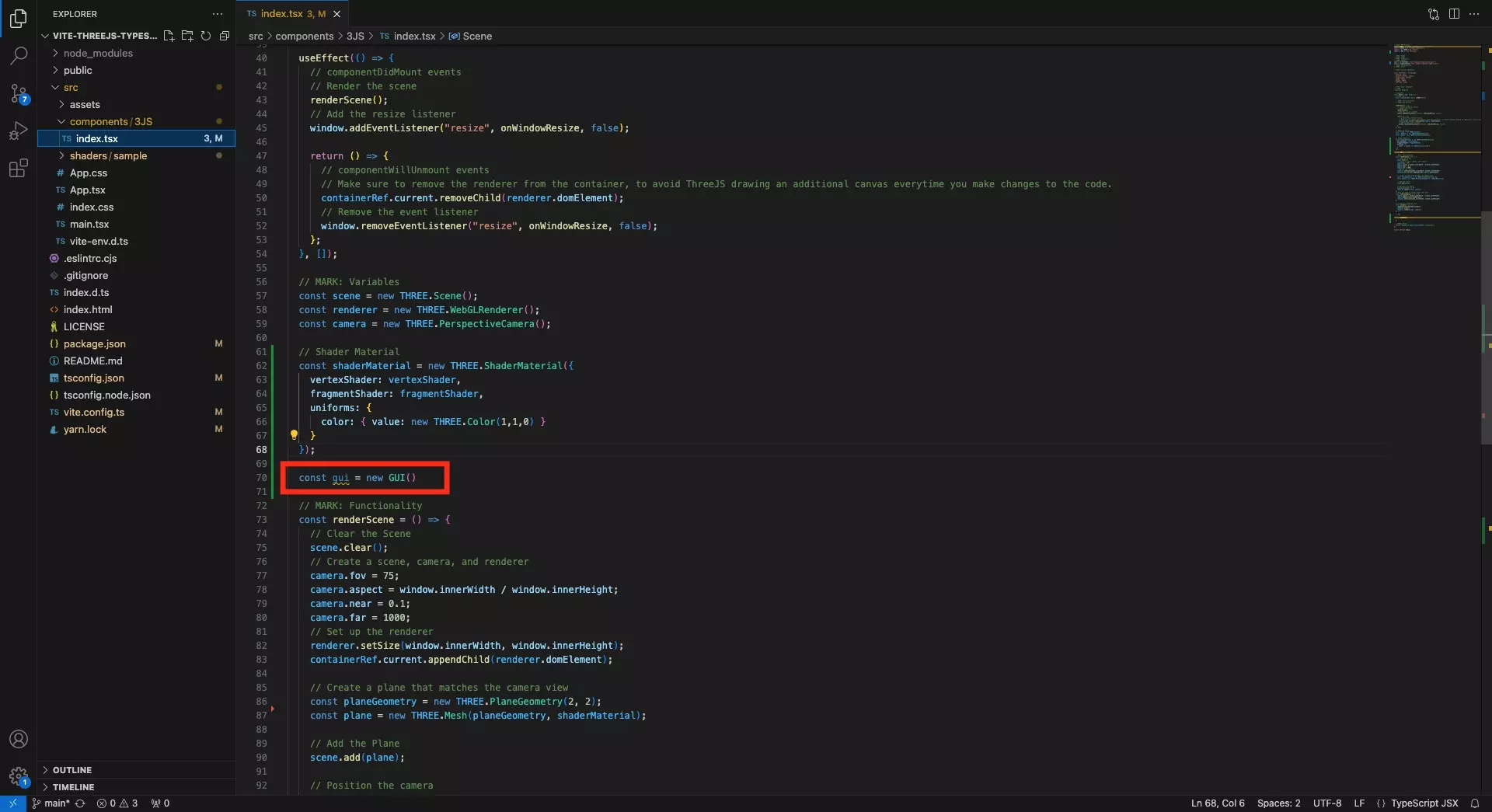
Add a new GUI constant to add the base controls to the scene.
const gui = new GUI()
Step Five: Setup the GUI
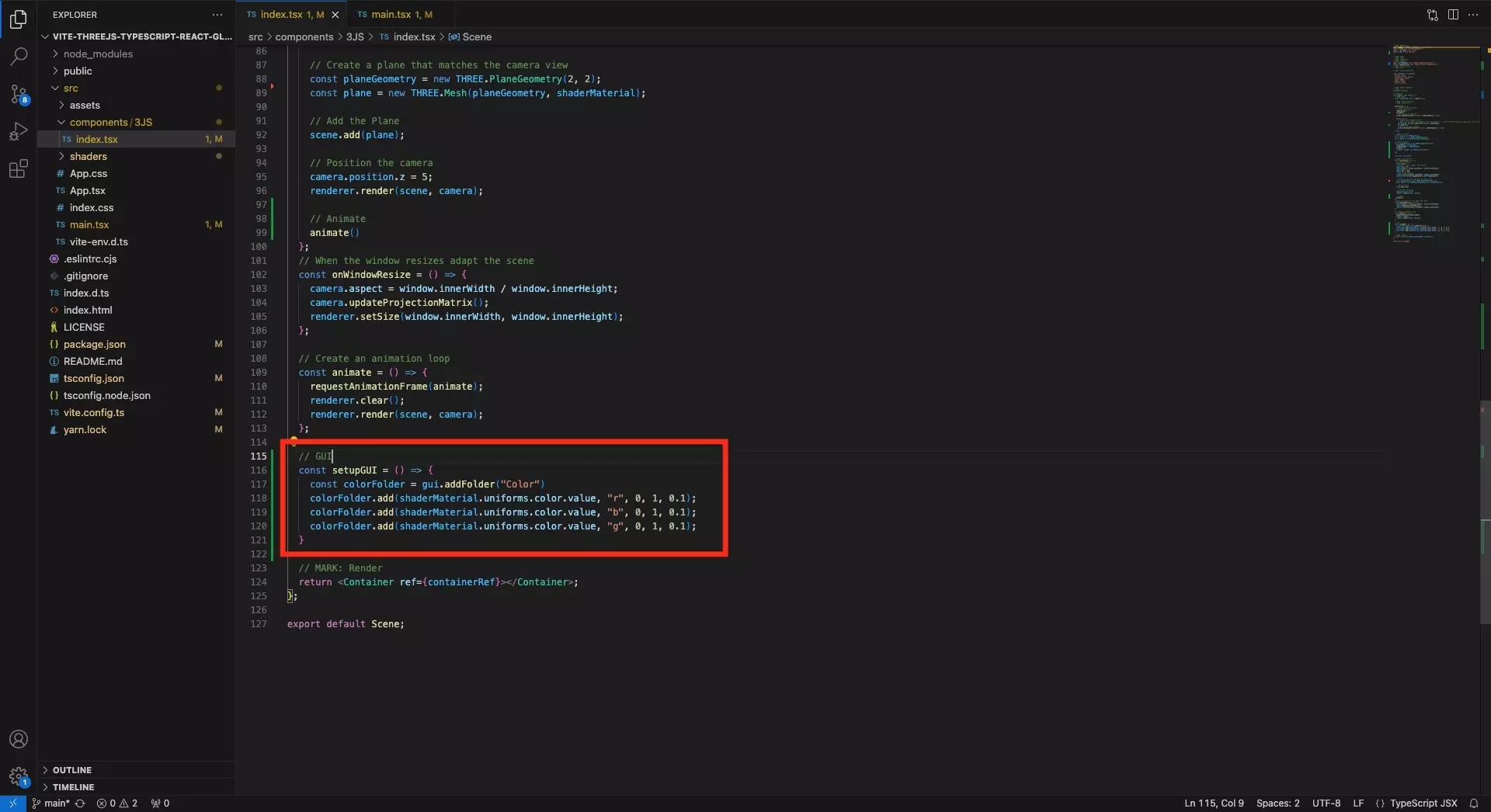
Create a function that dictates what functionality the GUI has.
Step Six: Update UseEffect
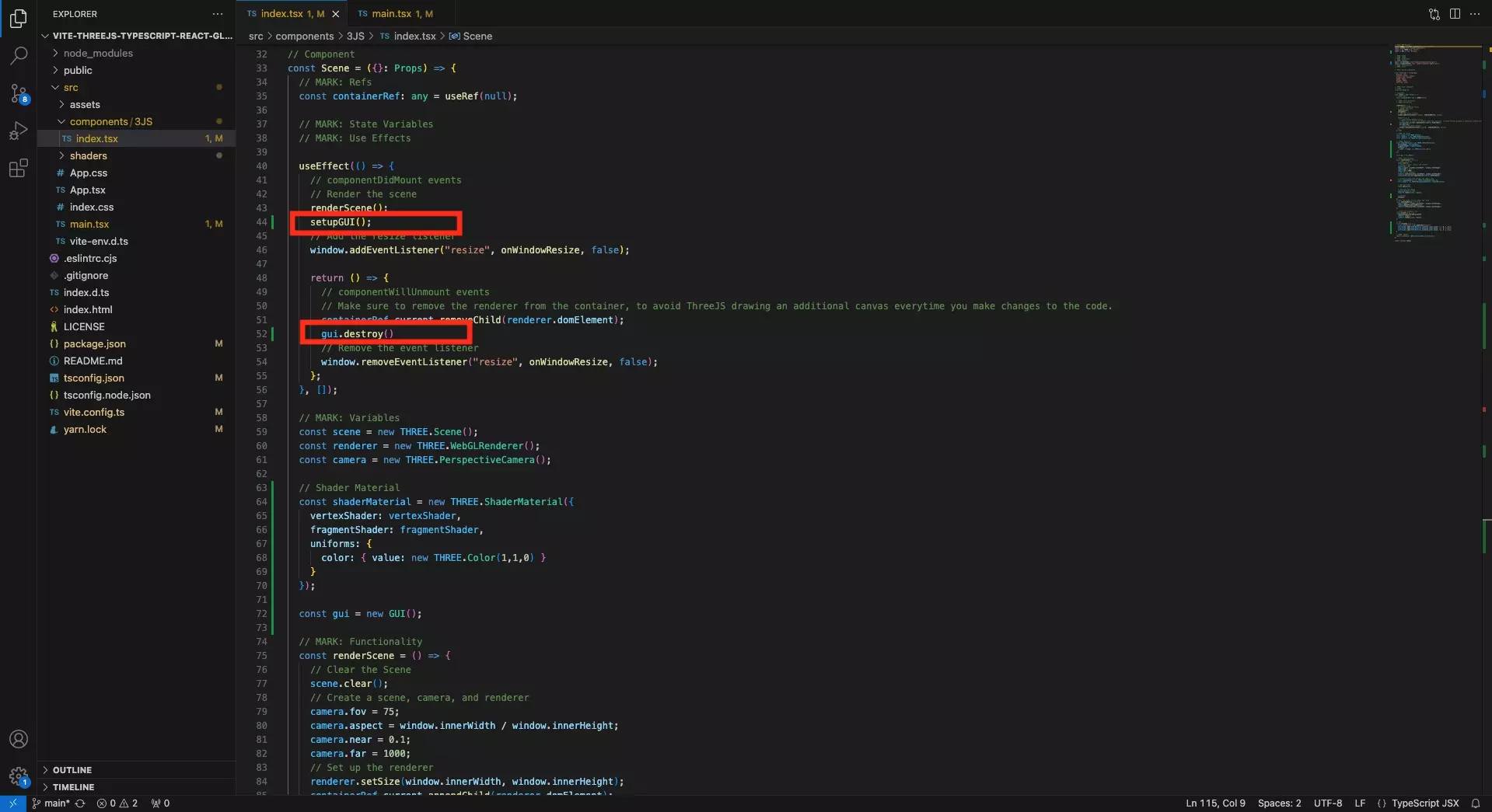
Update the useEffect function to setup the GUI on load and destroy it on hot reload.
If you do not do this, you will either see multiple GUI or see errors that pertain to folders already existing.
Step Seven: Remove Strict Mode
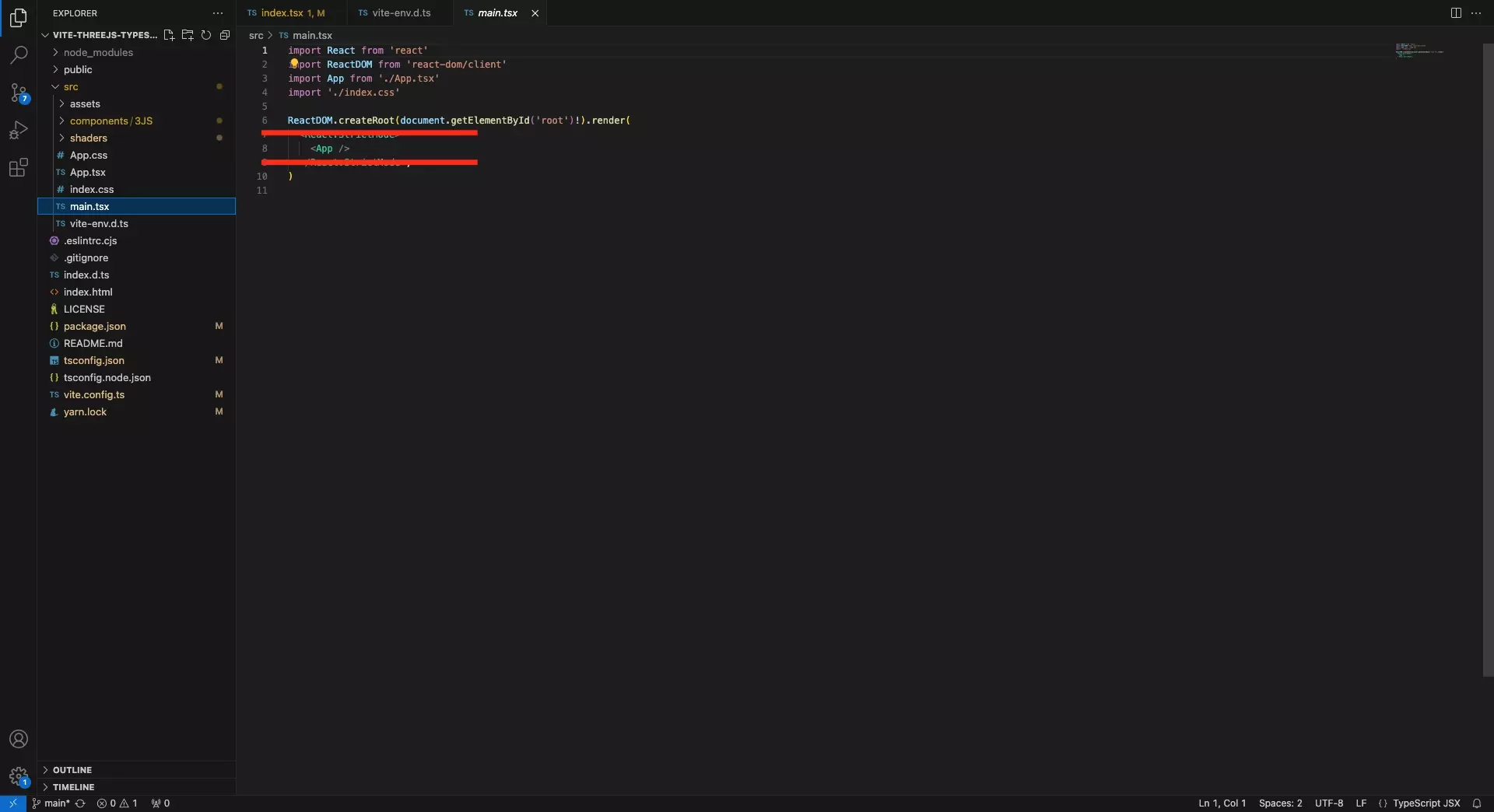
For dat.gui to work, you must remove the <React.StrictMode> from the file that links to the app.tsx.
In ViteJS this is the main.tsx, in ReactJS this is the index.tsx.
Step Eight: Test
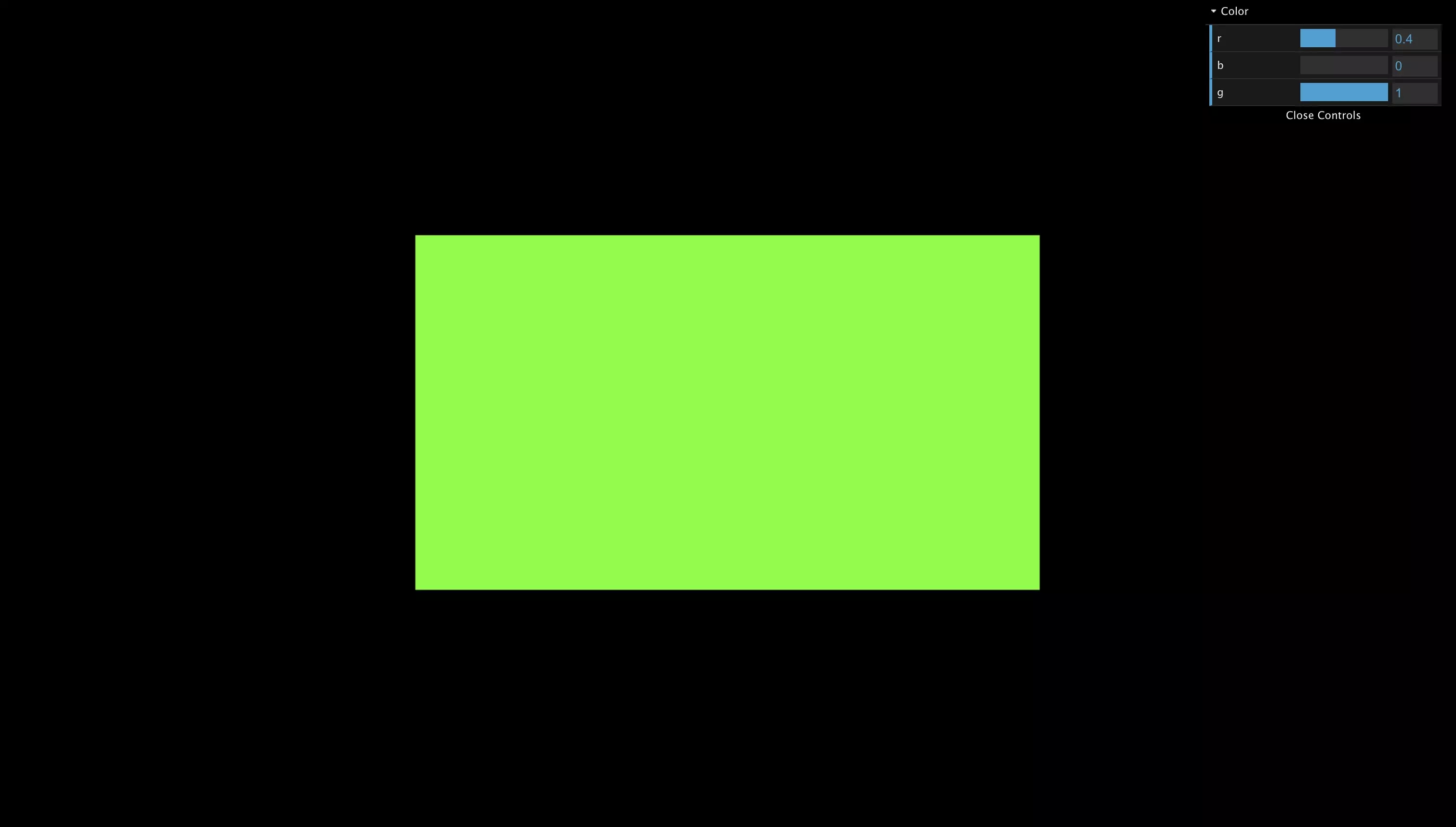
Run the app and confirm that you can dynamically change the shader.
Looking to learn more about ReactJS and ThreeJS ?
Search our blog to find educational content on learning how to use ReactJS and ThreeJS.